Storing Secrets
How to store secrets and environment variables in Beam
Managing Secrets and Environment Variables
In the Beam dashboard, you’ll be able to create secrets and environment variables which you can access in your apps.
Secrets can be scoped globally to all of your apps, or limited to a specific app.
Using Secrets
Once created, you can access a secret like an environment variable:
import os
my_secret_key = os.environ['MY_SECRET_KEY']
Creating a globally accessible secret
To create a new secret that is available across all apps in your Beam account, navigate to the Secrets Manager and click Create Secret.
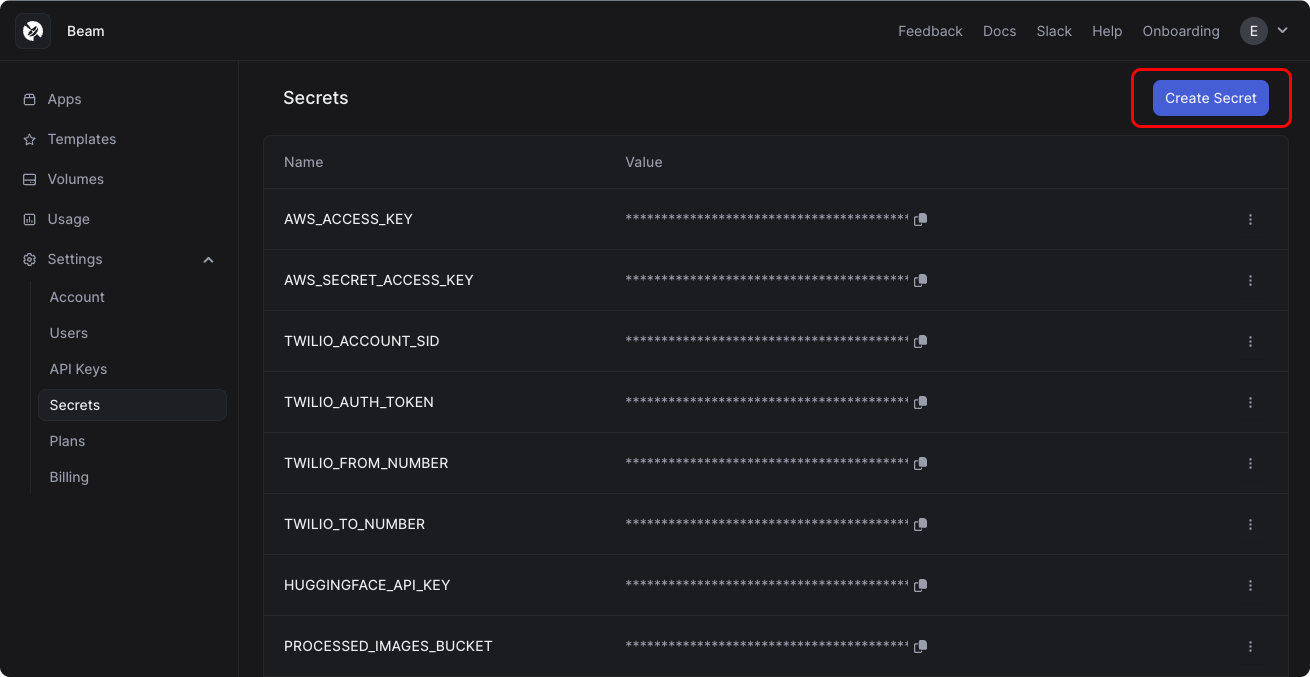
You’ll be prompted to enter a name and value.
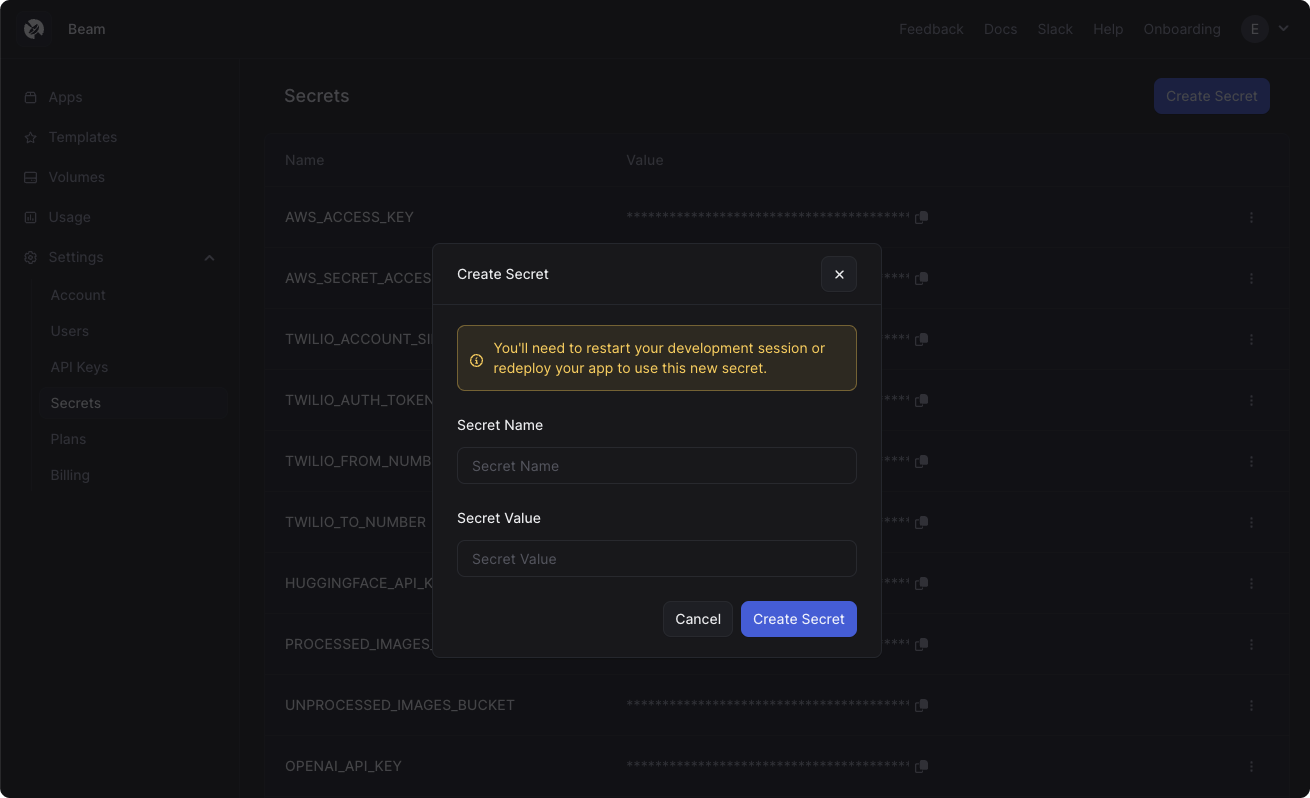
Creating an app-level secret
You can also create a secret that is only available for a specific app. To create an app-level secret: click an App
-> App Settings
-> Secrets
.
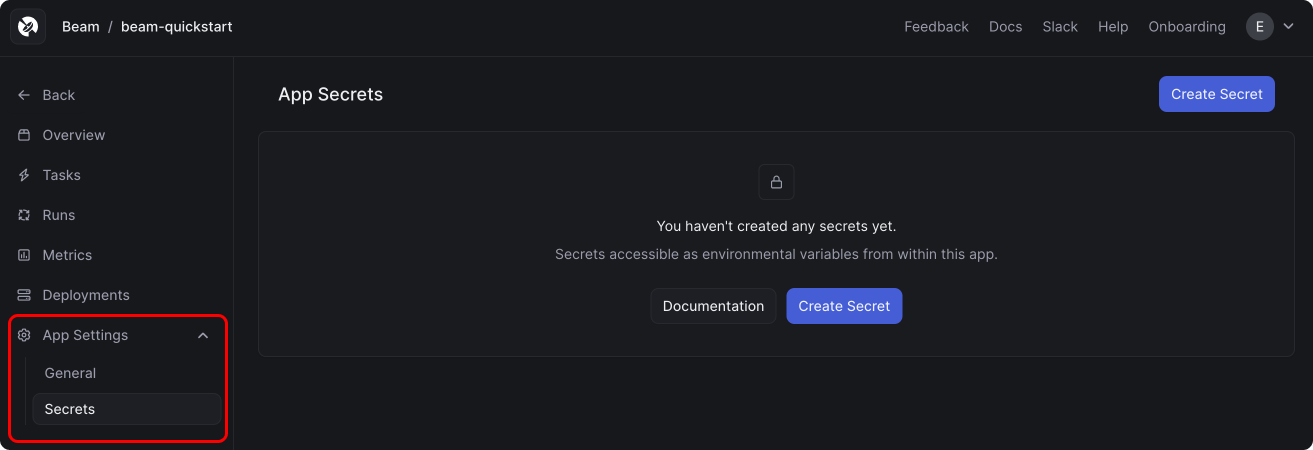
Loading secrets from your local environment
You might want to load secrets from a local .env
file. Beam includes an SDK method called Image.get_credentials_from_env()
which will load environment variables from a local .env
file into the remote Beam environment.
In the example below, we’ll pass AWS credentials from a local .env
file to Beam:
from beam import App, Image, Runtime
app = App(
name="load-secrets",
runtime=Runtime(
image=Image(
# Import base image from private ECR registry
base_image="683656326992.dkr.ecr.us-east-1.amazonaws.com/beam-test",
# Retrieve secrets from local .env file
base_image_creds=Image.get_credentials_from_env(
["AWS_ACCESS_KEY", "AWS_SECRET_KEY", "AWS_REGION"]
),
)
),
)
The .env
file should look like this:
#!/bin/bash
export AWS_REGION=YOUR_REGION
export AWS_SECRET_KEY=YOUR_KEY
export AWS_ACCESS_KEY=YOUR_KEY
Make sure to source the .env file before running beam deploy [app].py
Was this page helpful?