Calling the API
After deploying an app, you’ll be able to copy a cURL request to hit the API. In your app dashboard, click Call API.
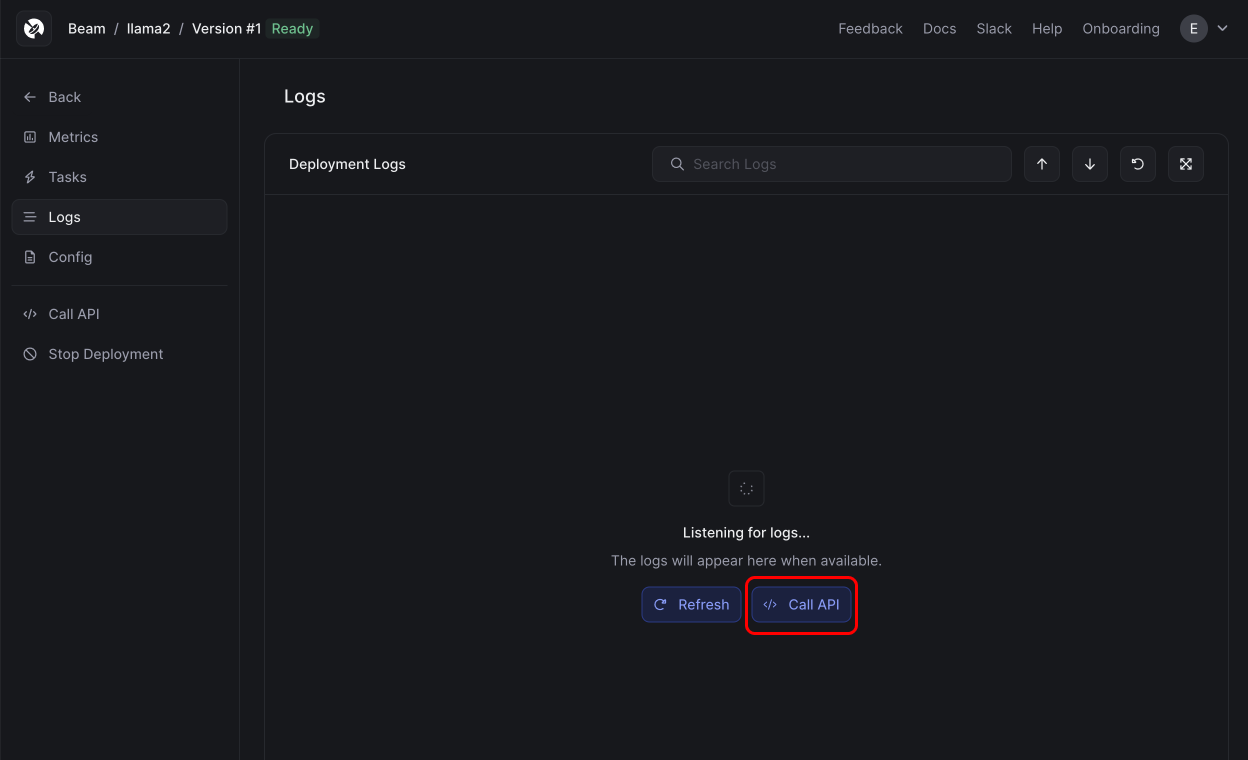
Here’s what a typical request to a REST API deployed on Beam might look like:
curl -X POST --compressed "https://apps.beam.cloud/0uc7x" \
-H 'Accept: */*' \
-H 'Accept-Encoding: gzip, deflate' \
-H 'Authorization: Basic [YOUR_AUTH_TOKEN]' \
-H 'Connection: keep-alive' \
-H 'Content-Type: application/json' \
-d '{"text": "This is an example request!"}'
Authentication
API Keys
Your APIs are authenticated through Basic Authentication. This takes the form of a base64-encoded client ID and client secret, joined by a colon:
client_id:client_secret
Your client_id
and client_secret
can be found in the API Keys section of the dashboard.
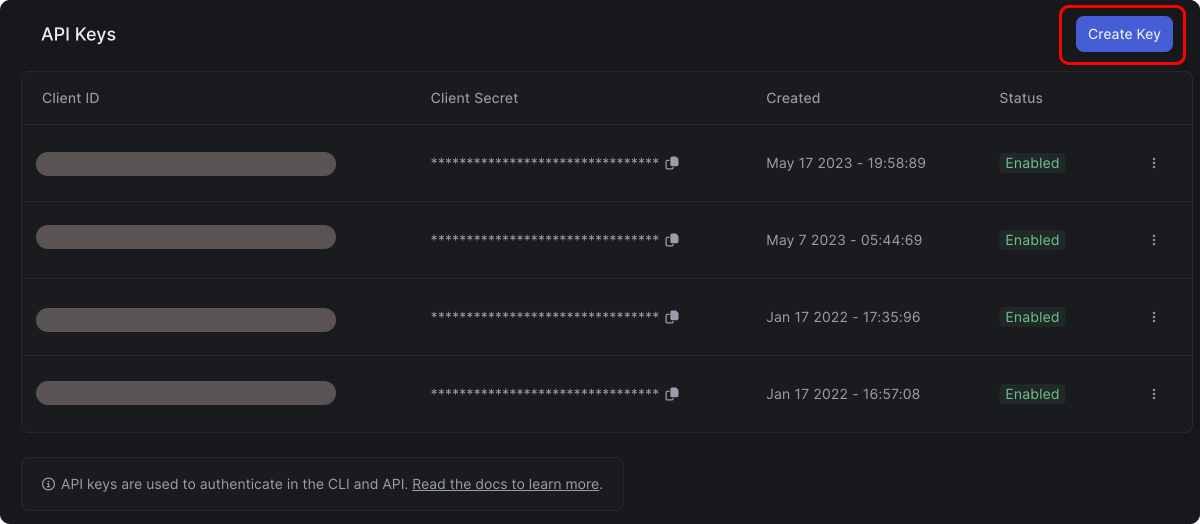
Encoding auth tokens
You can encode the auth tokens yourself, or copy a code-snippet generated for you in the dashboard.
Authenticating requests
A proper auth header will look like this:
Authorization: Basic ZGVtbzpwQDU1dzByZA==
And an authenticated cURL request will look something like:
curl -X POST --compressed "https://apps.beam.cloud/kajru" \
-H 'Authorization: Basic [YOUR_AUTH_TOKEN]'
Response codes
Beam uses standard HTTP codes to indicate the success or failure of your requests.
In general, 2xx
HTTP codes correspond to success, 4xx
codes are for user-related failures, and 5xx
codes are for infrastructure issues.
Status | Description |
---|---|
200 | Successful request. |
400 | Check that the parameters were correct. |
401 | The auth token used was invalid. |
5xx | Indicates an API timeout or an error with Beam servers. |
Custom response codes
You might want to return special status codes from your REST API. Beam supports FastAPI responses, so you can return custom status codes.
For example, you could return a 404
status code with a custom message in your handler
function:
from fastapi import FastAPI
from fastapi.responses import JSONResponse
def custom_api_response():
return JSONResponse(
status_code=404,
content={"message": "You need more tokens"}
)
Was this page helpful?