Storage Volumes
Using Volumes to manage data in Beam
Beam allows you to create highly-available storage volumes that can be used across tasks. You might use volumes for things like storing model weights or large datasets.
Mounting a Volume
Volumes can be attached to an App. By default, Volumes are shared across all apps in your Beam account.
from beam import App, Volume
app = App(
name="my_app",
volumes=[
Volume(
name="my_models",
path="models",
)
],
)
You can also restrict a volume to a specific app by specifying a VolumeType
when your volume is registered to an app: volume_type=VolumeType.Persistent
from beam import App, Volume, VolumeType
app = App(
name="my_app",
volumes=[Volume(name="my_models", path="models", VolumeType=VolumeType.Persistent)],
)
Reading and writing to volumes
You can read and write to your Volume like any ordinary Python file:
from beam import App, Volume
volume_path = "./models"
app = App(
name="my_app",
volumes=[
Volume(
name="my_models",
path=volume_path,
)
],
)
@app.run()
def write_files():
with open(f"{volume_path}/somefile.txt", "w") as f:
f.write("Writing to the volume!")
@app.run()
def read_files():
with open(f"{volume_path}/somefile.txt", "r") as f:
f.read()
Uploading Files with the CLI
You can upload files with the Beam CLI, for example:
beam volume upload [volume name] [file path] -a [app name]
If uploading to a Persistent Volume type, the app name must be provided using the -a
flag:
beam volume upload my_volume some_file.png -a the_app
If uploading to a Shared Volume, the app flag can be excluded.
Uploading Files with the Dashboard
You can also upload files with the web dashboard, on the volumes page.
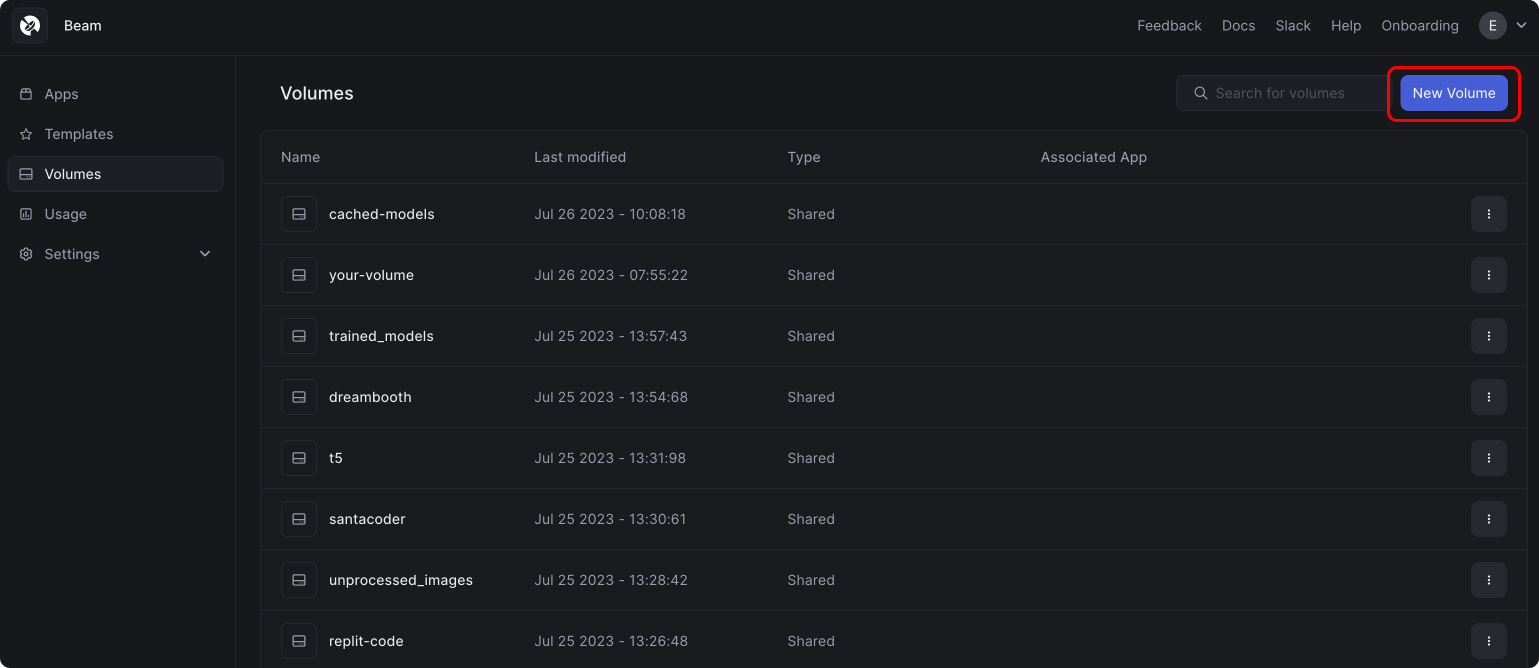
Uploading Folders Recursively in Python
You can recursively upload folders through the CLI, or through a Python script:
First Step
Copy the code below to a file on your local machine. Save the file as upload.py
.
Second Step
Run this command:
beam run upload.py:upload -d '{"local_path": "./YOUR_DIR", "beam_volume_path": "./YOUR_BEAM_VOLUME"}'
Third Step
Run beam volume ls [YOUR_VOLUME_NAME]
to verify that your files were uploaded!
Browsing Volume Contents
You can list all your volumes through the web dashboard, or CLI using the beam volume list
command.
You can also browse all contents of a volume with the beam volume ls
command:
Deleting Volumes
You can delete a volume in the web dashboard. Click the three-dots icon next to a volume, and click Delete Volume
to permanently delete it.
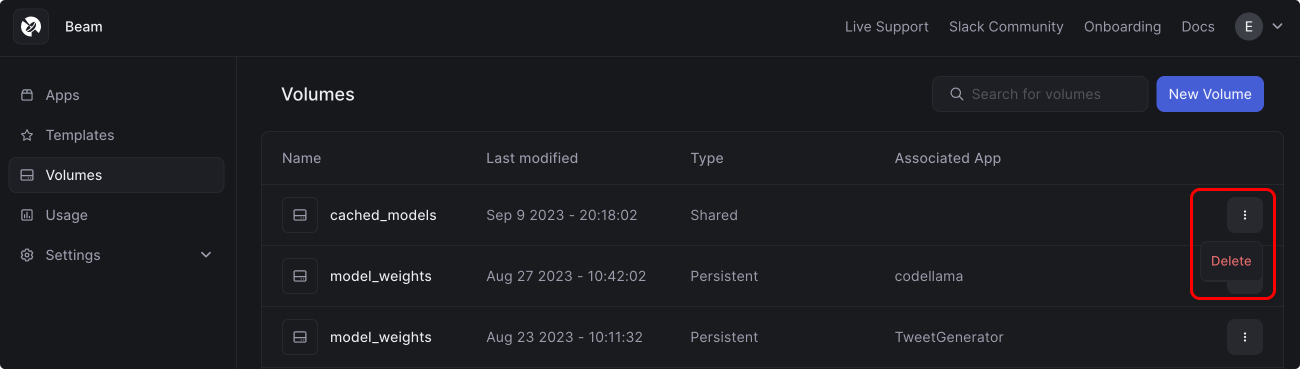
Was this page helpful?