July 2, 2024
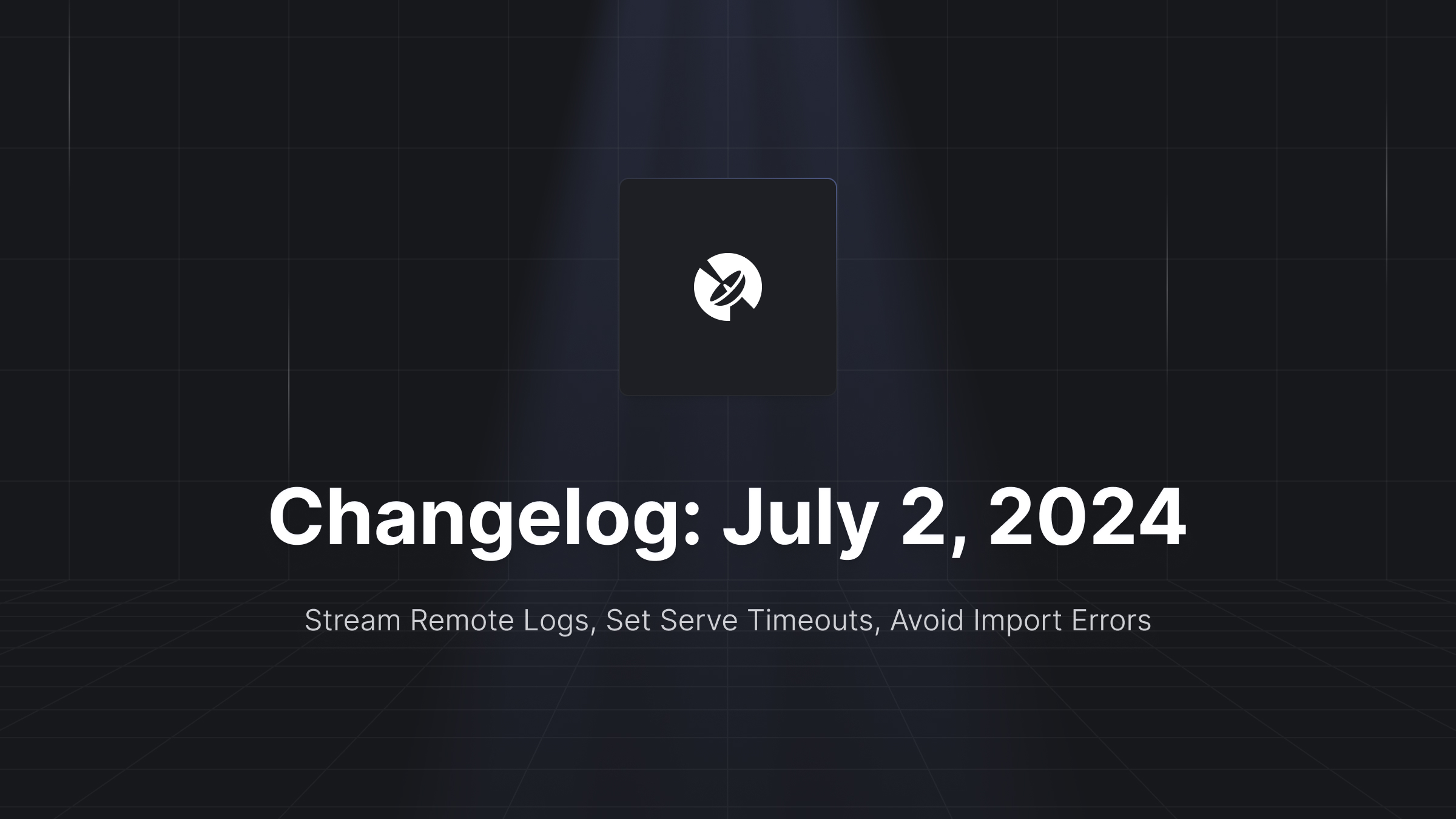
0.2.43
- Added
beam logs
command to stream logs to a local shell - Added custom timeouts for serves
- Added
env.is_remote()
to avoid import errors when running Beam apps locally - Signup for Beam V2 without a waitlist
Upgrade to the latest CLI:
Stream Remote Logs with beam logs
To simplify remote debugging, you can now stream logs from a task, deployment, or a container directly to your shell.
Deployment
Streams logs for a deployment.
You can find the deployment ID by running beam deployment list
.
Task
Streams logs for a task.
You can find the task ID by running beam task list
.
Container
Streams logs for a container.
You can find the container ID by running beam container list
.
Set Custom Timeouts for beam serve
You can configure how long to keep your serve session connected with the --timeout
flag.
- Timeout value of 0 will use the default timeout
- Timeout value of -1 will remove the timeout and persist the container for as long as the user is connected
Manage Remote Imports with env.is_remote()
Typically, your apps that run on Beam will be using packages that you don’t have installed locally.
If your Beam app uses packages that aren’t installed locally, you’ll need to ensure your Python interpreter doesn’t try to load these packages locally.
Avoiding Import Errors
There are two ways to avoid import errors when using packages that aren’t installed locally.
Import Packages Inline
Importing packages inline is safe because the functions will only be invoked in the remote Beam environment that has these packages installed.
Use env.is_remote()
An alternative to using inline imports is to use a special check called env.is_remote()
to conditionally import packages only when inside the remote environment.
This command checks whether the Python script is running remotely on Beam, and will only try to import the packages in its scope if it is.
While it might be tempting to use the env.is_remote()
flag for other logic
in your app, this command should only be used for package imports.
Bug Fixes
- Encrypted secret values no longer returned in
/task
status API - Fix nested list serialization in
**inputs
Was this page helpful?