Generating 3D Point Clouds
Using OpenAI’s Point-E to generate 3D point clouds
In this example, we’ll demonstrate using Beam to deploy a cloud endpoint for OpenAI’s Point-E, a state-of-the-art model for generating 3D objects.
Setting up the environment
First, you’ll setup your compute environment. You’ll specify:
- Compute requirements
- Python packages to install in the runtime
from beam import App, Runtime, Image, Output, Volume
app = App(
name="pointe",
runtime=Runtime(
cpu=1,
memory="8Gi",
gpu="A10G",
image=Image(
python_packages=[
"filelock",
"Pillow",
"torch",
"fire",
"humanize",
"requests",
"tqdm",
"matplotlib",
"scikit-image",
"scipy",
"numpy",
"clip@git+https://github.com/openai/CLIP.git",
],
),
),
)
Generating point clouds
Make sure you’ve cloned the point-e repo
into your working directory — otherwise, the point_e
imports won’t resolve.
This code is copied directly from this example notebook in the Point-E repo.
We’ve only made two modifications:
- Accept by adding
**inputs
to the function parameters - Replacing the hardcoded prompt with a dynamic prompt you’ll pass down from the API, as
inputs["prompt"]
Deployment
You’ll deploy the app by entering your shell, and running:
beam deploy app.py
Calling the API
If you navigate to the link in the last line of the shell output, you’ll be able to login to your Beam web dashboard and view the details of your deployment.
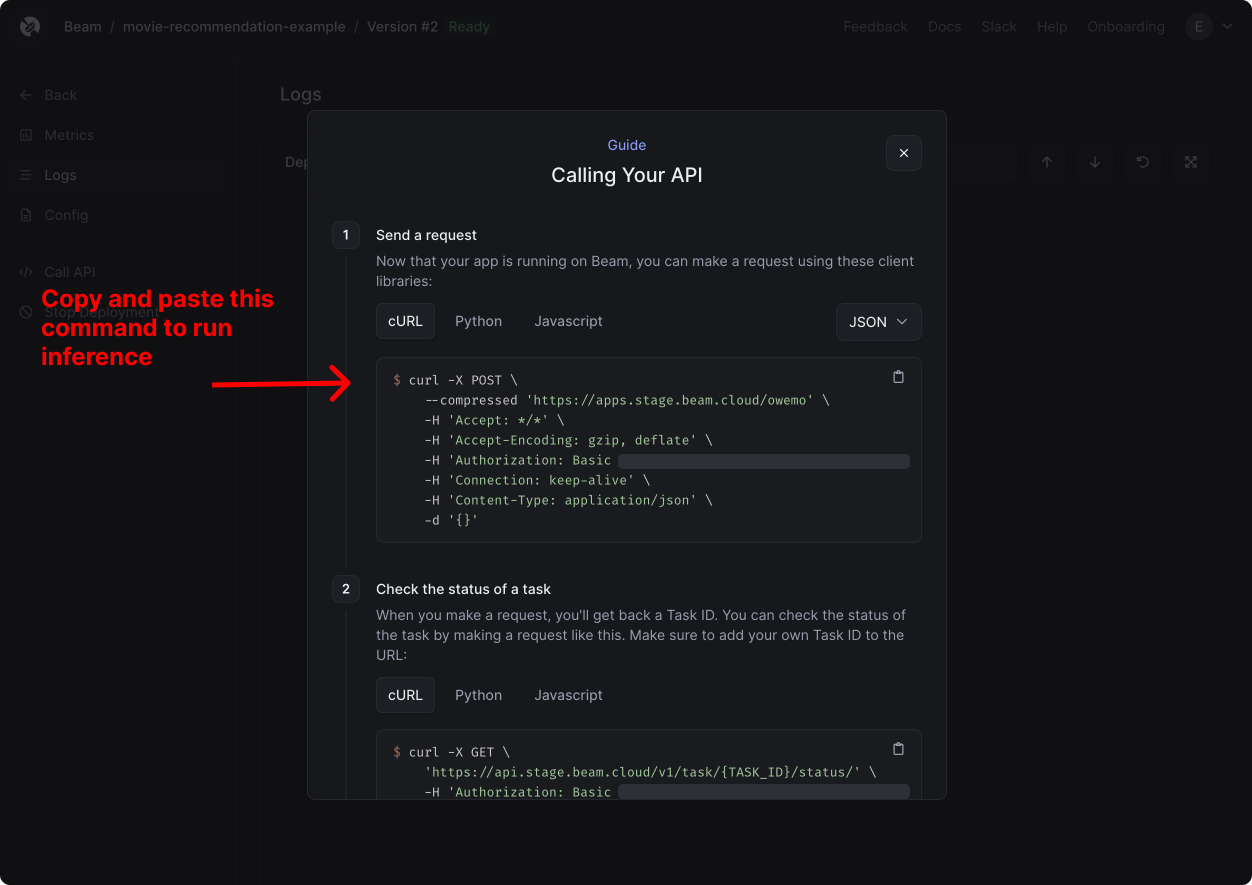
You’ll call the API by pasting in the cURL command displayed in the browser window.
curl -X POST --compressed "https://apps.beam.cloud/77n0o" \
-H 'Authorization: [YOUR_AUTH_TOKEN]' \
-H 'Content-Type: application/json' \
-d '{"prompt": "a bicycle"}'
Since you’ve deployed a task queue, the API will return a Task ID.
{"task_id":"774cb94c-05ff-488e-80ab-58a239ce347d"}
Retrieving outputs
Because this function generates a file output, you’ll need to retrieve it. There are two ways to retrieve outputs:
- Logging into the dashboard, and viewing the tasks
- In the API, by querying the
/task
endpoint.
In the dashboard, you’ll see your output file appear in the Outputs table when the task is completed.
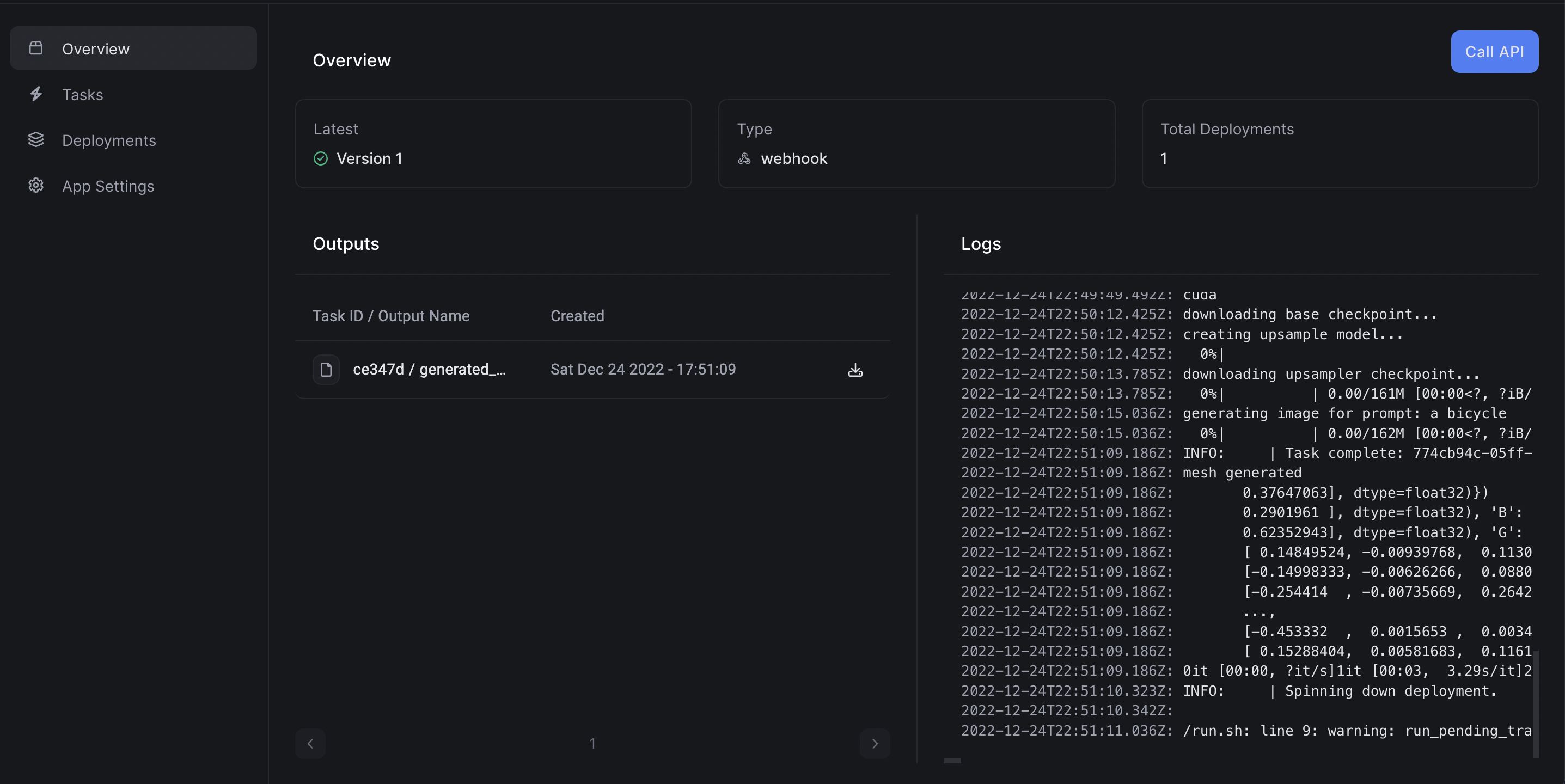
Click the Download icon, and open the file in a 3D viewing software. You’ll see a 3D point cloud generated from your prompt:
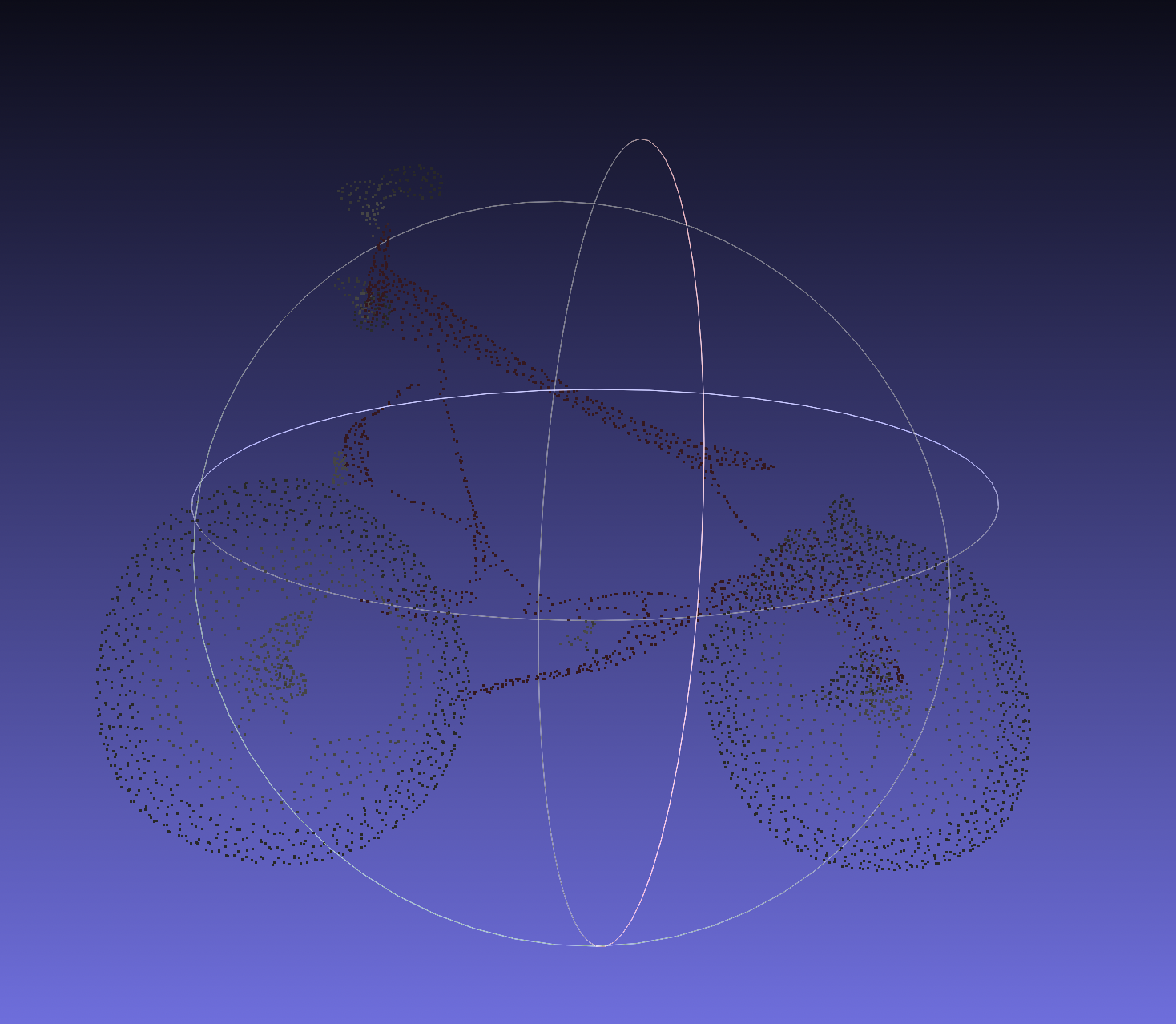
Was this page helpful?